Loading... ### meave配置(pom.xml) ```java <?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.example</groupId> <artifactId>TomcatMaven</artifactId> <version>1.0-SNAPSHOT</version> <dependencies> <dependency> <groupId>javax.servlet</groupId> <artifactId>javax.servlet-api</artifactId> <version>3.1.0</version> <scope>provided</scope> <!--注意一定不能少这里,否则会冲突--> </dependency> <dependency> <groupId>commons-io</groupId> <artifactId>commons-io</artifactId> <version>2.6</version> </dependency> <!--mybatis 依赖--> <dependency> <groupId>org.mybatis</groupId> <artifactId>mybatis</artifactId> <version>3.5.5</version> </dependency> <!--mysql 驱动--> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> <version>8.0.29</version> </dependency> <!--jsp--> <dependency> <groupId>javax.servlet.jsp</groupId> <artifactId>jsp-api</artifactId> <version>2.2</version> <scope>provided</scope> </dependency> <!--jstl--> <dependency> <groupId>jstl</groupId> <artifactId>jstl</artifactId> <version>1.2</version> </dependency> <dependency> <groupId>taglibs</groupId> <artifactId>standard</artifactId> <version>1.1.2</version> </dependency> <dependency> <groupId>com.alibaba</groupId> <artifactId>fastjson</artifactId> <version>2.0.7.graal</version> </dependency> <dependency> <groupId>org.junit.jupiter</groupId> <artifactId>junit-jupiter</artifactId> <version>RELEASE</version> <scope>compile</scope> </dependency> </dependencies> <!-- tomcat 插件 --> <build> <plugins> <plugin> <groupId>org.apache.tomcat.maven</groupId> <artifactId>tomcat7-maven-plugin</artifactId> <version>2.2</version> <configuration> <port>80</port> <path>/</path> </configuration> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <configuration> <source>7</source> <target>7</target> </configuration> </plugin> </plugins> </build> <!-- <packaging>:打包方式 :jar:默认值 :war:web项目 --> <packaging>war</packaging> </project> ``` ## mybatis配置(mybatis-config.xml) ```java <?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <!--起别名--> <typeAliases> <package name="com.pojo"/> </typeAliases> <environments default="development"> <environment id="development"> <transactionManager type="JDBC"/> <dataSource type="POOLED"> <property name="driver" value="com.mysql.jdbc.Driver"/> <property name="url" value="jdbc:mysql:///mybatis?useSSL=false&useServerPrepStmts=true"/> <property name="username" value="root"/> <property name="password" value="Abc12345678"/> </dataSource> </environment> </environments> <mappers> <!--扫描mapper--> <package name="com.mapper"/> </mappers> </configuration> ``` ## 目录一般结构 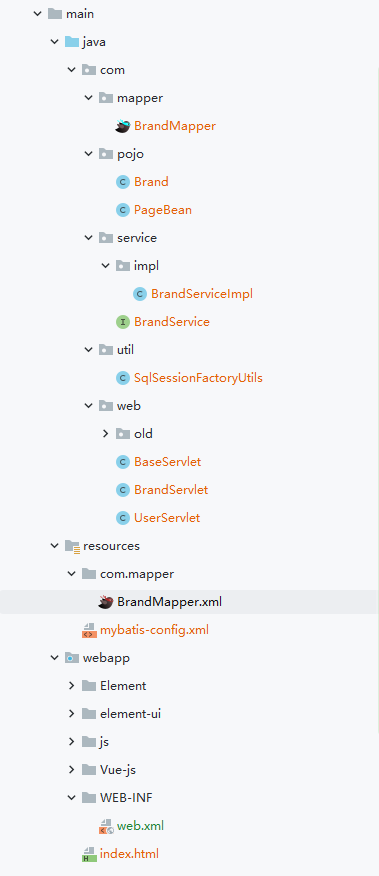 ## SqlSessionFactoryUtil工具包 ```java package com.util; import org.apache.ibatis.io.Resources; import org.apache.ibatis.session.SqlSessionFactory; import org.apache.ibatis.session.SqlSessionFactoryBuilder; import java.io.IOException; import java.io.InputStream; public class SqlSessionFactoryUtils { private static SqlSessionFactory sqlSessionFactory; static { //静态代码块会随着类的加载而自动执行,且只执行一次 try { String resource = "mybatis-config.xml"; InputStream inputStream = Resources.getResourceAsStream(resource); sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream); } catch (IOException e) { e.printStackTrace(); } } public static SqlSessionFactory getSqlSessionFactory(){ return sqlSessionFactory; } } ``` ## BrandMapper.xml ```java <?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <mapper namespace="com.mapper.BrandMapper"> <resultMap id="brandResultMap" type="brand"> <result property="brandName" column="brand_name" /> <result property="companyName" column="company_name" /> </resultMap> <update id="update"> update tb_brand set brand_name = #{brand.brandName}, company_name = #{brand.companyName}, ordered = #{brand.ordered}, description = #{brand.description}, status = #{brand.status} where id = #{brand.id} </update> <delete id="deleteByIds" > delete from tb_brand where id in <foreach collection="ids" item="id" separator="," open="(" close=")"> #{id} </foreach> </delete> <select id="selectByPageAndCondition" resultMap="brandResultMap"> select * from tb_brand <where> <if test="brand.brandName != null and brand.brandName != ''"> and brand_name like #{brand.brandName} </if> <if test="brand.companyName != null and brand.companyName != ''"> and company_name like #{brand.companyName} </if> <if test="brand.status != null"> and status = #{brand.status} </if> </where> limit #{begin}, #{size} </select> <select id="selectTotalCountByCondition" resultType="java.lang.Integer"> select count(*) from tb_brand <where> <if test="brandName != null and brandName != ''"> and brand_name like #{brandName} </if> <if test="companyName != null and companyName != ''"> and company_name like #{companyName} </if> <if test="status != null"> and status = #{status} </if> </where> </select> </mapper> ``` ## BaseServlet 我们可以通过BaseServlet替换HttpServlet, 根据请求的最后一段路径来进行分发请求 ```java package com.web; /** * @code Description * @code author 本当迷 * @code date 2022/7/24-20:24 */ import javax.servlet.ServletException; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.IOException; import java.lang.reflect.InvocationTargetException; import java.lang.reflect.Method; /** * 替换HttpServlet, 根据请求的最后一段路径来进行分发 */ public class BaseServlet extends HttpServlet { // 根据请求的最后一段路径来进行分发 @Override protected void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { // 1.获取请求路径 final String uri = req.getRequestURI(); // 2.获取最后一段路径,方法名 final int index = uri.lastIndexOf('/'); final String methodName = uri.substring(index + 1); // 3.执行方法 // 3.1获取BrandServlet / UserServlet 字节码对象 Class final Class<? extends BaseServlet> aClass = this.getClass(); // 3.3获取方法Method对象 try { final Method method = aClass.getMethod(methodName, HttpServletRequest.class, HttpServletResponse.class); // 3.4执行方法 method.invoke(this, req, resp); } catch (NoSuchMethodException | InvocationTargetException | IllegalAccessException e) { throw new RuntimeException(e); } } } ``` ## BrandMapper SQL处理 ```java package com.mapper; import com.pojo.Brand; import org.apache.ibatis.annotations.*; import java.util.List; public interface BrandMapper { /** * 查询所有 * @return */ @Select("select * from tb_brand") @ResultMap("brandResultMap") List<Brand> selectAll(); /** * 添加 * @param brand */ @Insert("insert into tb_brand values(null,#{brandName},#{companyName},#{ordered},#{description},#{status})") void add(Brand brand); /** * 批量删除 * @param ids */ void deleteByIds(@Param("ids") int[] ids); /** * 分页查询 * @param begin * @param size * @return */ @Select("select * from tb_brand limit #{begin}, #{size}") List<Brand> selectByPage(@Param("begin") int begin, @Param("size") int size); /** * 返回总页数 * @return */ @Select("select count(*) from tb_brand") int selectTotalCount(); /** * 分页条件查询 含模糊查询 * @param begin * @param size * @return */ List<Brand> selectByPageAndCondition(@Param("begin") int begin,@Param("size") int size,@Param("brand") Brand brand); /** * 根据条件查询总记录数 * @return */ int selectTotalCountByCondition(Brand brand); /** * 通过id修改对应信息 * @param brand 用户id */ void update(@Param("brand") Brand brand); } ``` ## BrandServlet增删改查 ```java package com.web; import com.alibaba.fastjson.JSON; import com.pojo.Brand; import com.pojo.PageBean; import com.service.BrandService; import com.service.impl.BrandServiceImpl; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import java.io.BufferedReader; import java.io.IOException; import java.util.Arrays; import java.util.List; /** * @code Description * @code author 本当迷 * @code date 2022/7/24-20:35 */ @WebServlet("/brand/*") public class BrandServlet extends BaseServlet{ private final BrandService brandService = new BrandServiceImpl(); /** * 查询所有数据 * @param request * @param response * @throws ServletException * @throws IOException */ public void selectAll(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { System.out.println("selectAll"); // 1.调用service查询 final List<Brand> brands = brandService.selectAll(); // 2.转为JSON final String jsonString = JSON.toJSONString(brands); // 3.写数据 response.setContentType("text/json;charset=utf-8"); response.getWriter().write(jsonString); } /** * 添加数据 * @param request * @param response * @throws ServletException * @throws IOException */ public void add(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { System.out.println("add"); // 1.接受品牌数据 final BufferedReader reader = request.getReader(); final String edit = request.getParameter("edit"); System.out.println(edit); // JSON数据 final String params = reader.readLine(); // 转为Brand对象 final Brand brand = JSON.parseObject(params, Brand.class); System.out.println(brand); if(edit.equals("false")){ // 2.调用service添加 brandService.add(brand); }else{ brandService.update(brand); } response.getWriter().write("success"); } /** * 批量删除 * @param request * @param response * @throws ServletException * @throws IOException */ public void deleteIds(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { System.out.println("deleteIdS"); // 1.接受品牌数据 final BufferedReader reader = request.getReader(); // JSON数据 final String params = reader.readLine(); // 转为Brand对象 final int[] ints = JSON.parseObject(params, int[].class); System.out.println(Arrays.toString(ints)); // 2.调用service添加 brandService.deleteByIds(ints); // 3.响应成功的信息 response.getWriter().write("success"); } /** * 分页查询 * @param request * @param response * @throws ServletException * @throws IOException */ public void selectByPage(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { // 1.接受 当前页码 和 每页展示条数 final int currentPage = Integer.parseInt(request.getParameter("currentPage")); final int pageSize = Integer.parseInt(request.getParameter("pageSize")); // 2.调用service查询 final PageBean<Brand> brandPageBean = brandService.selectByPage(currentPage, pageSize); // 2.转为JSON final String jsonString = JSON.toJSONString(brandPageBean); // 3.写数据 response.setContentType("text/json;charset=utf-8"); response.getWriter().write(jsonString); } /** * 分页条件查询 * @param request * @param response * @throws ServletException * @throws IOException */ public void selectByPageAndCondition(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { System.out.println("开始查询!——————————————————————————"); //1. 接收 当前页码 和 每页展示条数 url?currentPage=1&pageSize=5 String _currentPage = request.getParameter("currentPage"); String _pageSize = request.getParameter("pageSize"); int currentPage = Integer.parseInt(_currentPage); int pageSize = Integer.parseInt(_pageSize); // 获取查询条件对象 BufferedReader br = request.getReader(); String params = br.readLine();//json字符串 //转为 Brand Brand brand = JSON.parseObject(params, Brand.class); //2. 调用service查询 PageBean<Brand> pageBean = brandService.selectByPageAndCondition(currentPage,pageSize,brand); System.out.println(pageBean); //2. 转为JSON String jsonString = JSON.toJSONString(pageBean); //3. 写数据 response.setContentType("text/json;charset=utf-8"); response.getWriter().write(jsonString); } } ``` ## 完结 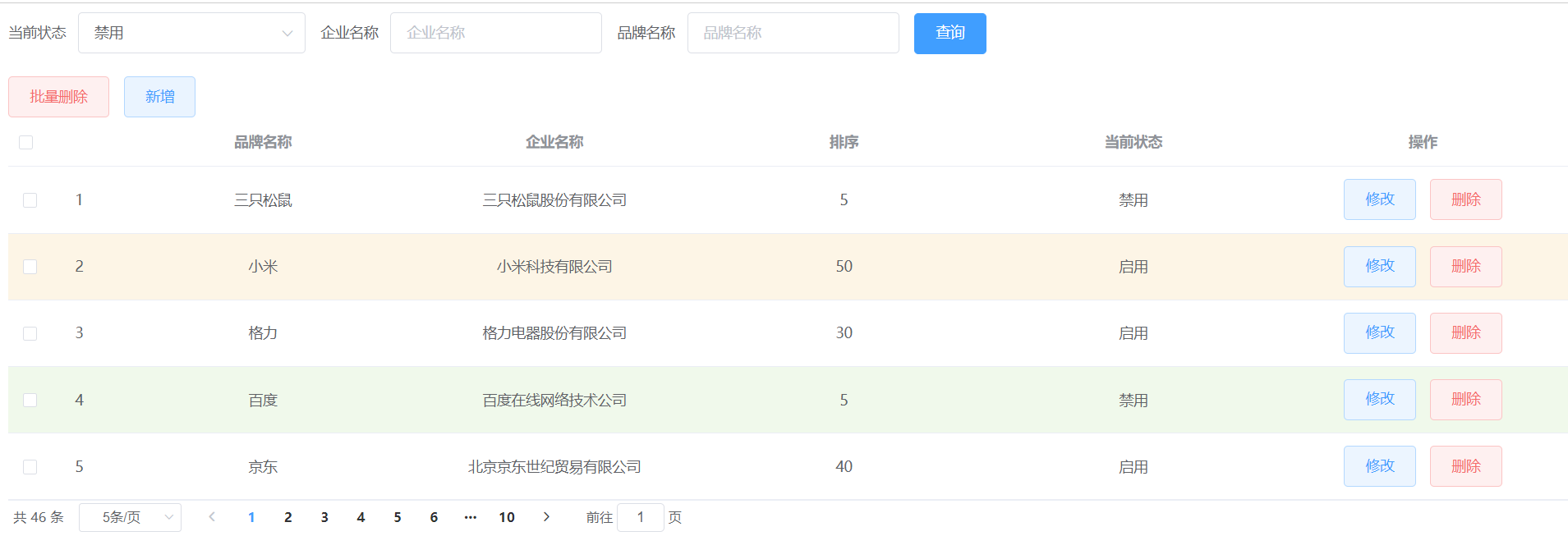 最后修改:2022 年 07 月 27 日 © 允许规范转载 打赏 赞赏作者 支付宝微信 赞 如果文章有用,请随意打赏。